What are Some Hidden Features of Python?
Python is an incredibly popular programming language known for its simplicity and readability. However, beyond its fundamental syntax and well-known features, Python also offers a range of hidden gems that can enhance your coding experience and productivity. In this article, we will explore some of these hidden features in Python that may have gone unnoticed.
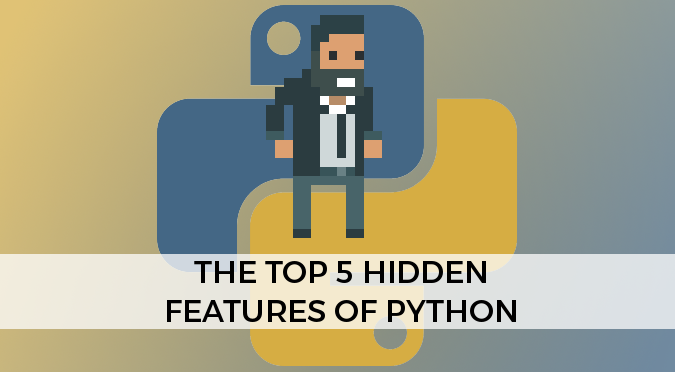
Credit: www.alanzucconi.com
1. List Comprehensions
List comprehensions allow you to create new lists based on existing lists, making code more concise and elegant. They follow a simple syntax:
[expression for item in iterable]
This creates a new list by applying the expression to each item in the iterable. For example:
numbers = [1, 2, 3, 4, 5]
squared = [x2 for x in numbers]
print(squared) # Output: [1, 4, 9, 16, 25]
2. Context Managers
Context managers provide a convenient way to manage resources, such as files or network connections, ensuring they are properly handled. Python offers a built-in with
statement that automatically handles the setup and teardown of resources. For example:
with open('myfile.txt', 'r') as file:
for line in file:
print(line)
In the above example, the with
statement guarantees that the file will be closed once the block is exited, even if an exception occurs. This helps prevent resource leaks and makes your code more robust.
3. Namedtuples
Namedtuples provide a convenient way to create lightweight objects with named fields, similar to a struct in C. Unlike regular tuples, namedtuples allow you to access fields using dot notation. They are defined using the collections.namedtuple
function. For example:
from collections import namedtuple
Person = namedtuple('Person', ['name', 'age'])
person = Person('John Doe', 30)
print(person.name, person.age) # Output: John Doe 30
4. Underscore as a Throwaway Variable
In Python, sometimes you need to use a variable in a loop or function, but you don’t actually care about its value. In such cases, you can use an underscore (_
) as a throwaway variable. It is a common convention to use _
for ignored values. For example:
for _ in range(10):
# Perform an action without using the loop variable
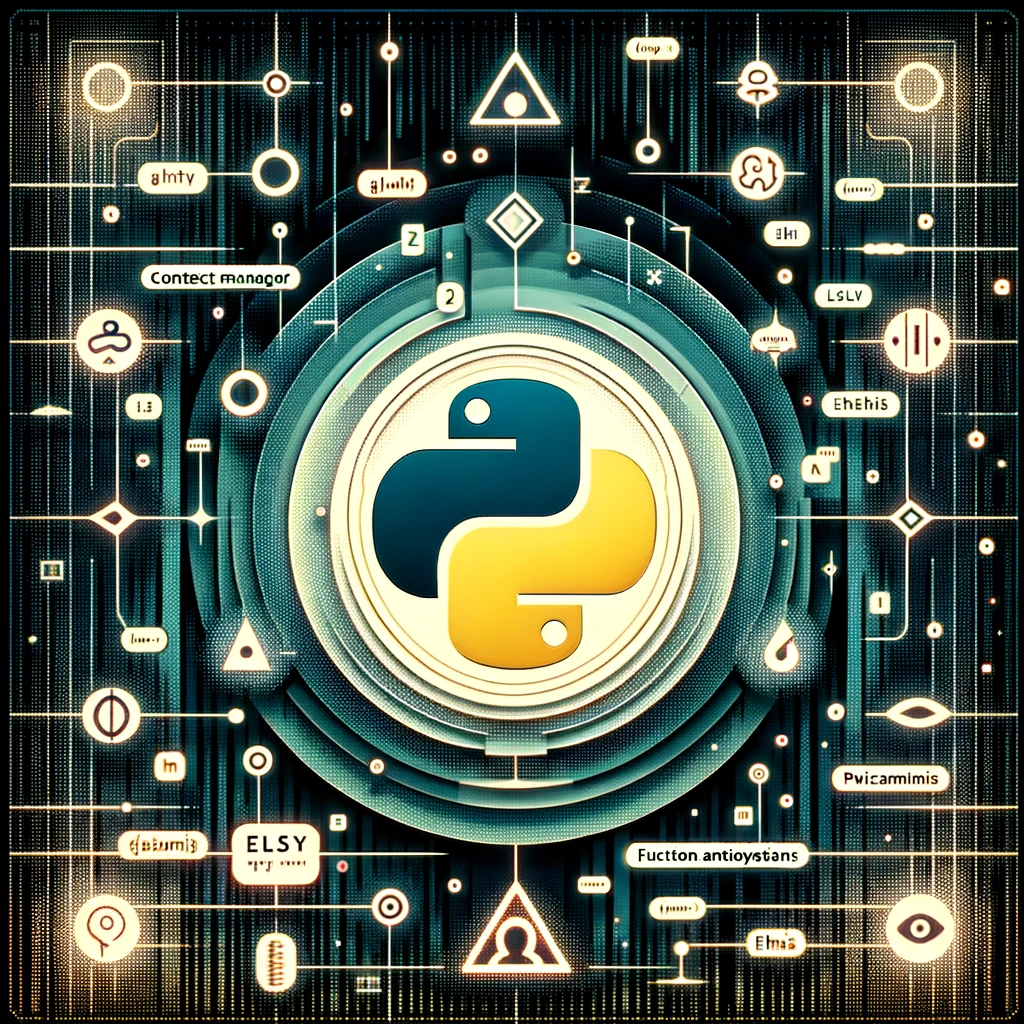
Credit: developer-service.blog
5. Extended Iterable Unpacking
Python allows you to unpack an iterable (e.g., a list or tuple) into multiple variables, making it easy to assign values returned by functions or iterate over collections. This feature is known as iterable unpacking. In Python 3, you can also use an asterisk () to capture the remaining elements into a separate variable. For example:
a, b, rest = [1, 2, 3, 4, 5]
print(a, b) # Output: 1 2
print(rest) # Output: [3, 4, 5]
Frequently Asked Questions For What Are Some Hidden Features Of Python?
What Are Some Hidden Features Of Python?
Python, being a versatile programming language, offers several hidden features that can enhance your coding experience.
How Can I Use Python’s List Comprehension Feature?
Python’s list comprehension feature allows you to create new lists in a concise and efficient manner, making your code more readable.
What Are Python Decorators And How Can They Be Used?
Python decorators are a powerful feature that enables you to modify the behavior of a function or class. They can be used to add functionality or modify existing code without changing the original codebase.
How Does Python’s Context Managers Help With Resource Management?
Python’s context managers, implemented through the `with` statement, provide an elegant way to handle resources such as files or network connections. They ensure that resources are properly acquired and released, even in case of exceptions.
Conclusion
Python is not only a simple and powerful programming language, but it also offers a range of hidden features that can make your coding experience even better. This article covered just a few of these hidden gems, including list comprehensions, context managers, namedtuples, underscore as a throwaway variable, and extended iterable unpacking. By exploring and utilizing these features, you can improve the readability, conciseness, and efficiency of your Python code.