Building a Doublylinkedlist in Python – – Append Method
In this article, we will explore how to build a doubly linked list in Python and focus on the append method. A doubly linked list is a data structure that consists of a sequence of nodes, where each node contains both the data and a reference to the next and previous nodes. This allows for efficient insertion and deletion operations at both the beginning and end of the list.
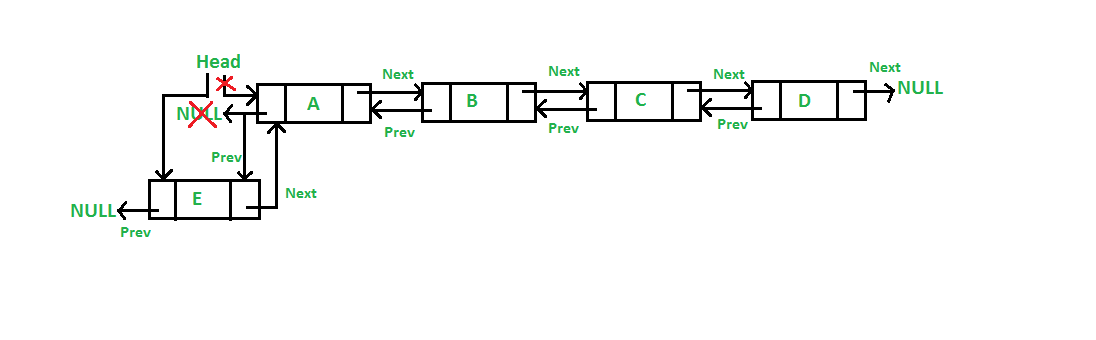
Credit: www.geeksforgeeks.org
What is a Doubly Linked List?
A doubly linked list is quite similar to a singly linked list, with the main difference being that each node in a doubly linked list contains a reference to both the next and previous nodes. This bidirectional connection between nodes provides added flexibility and allows for traversal in both directions.
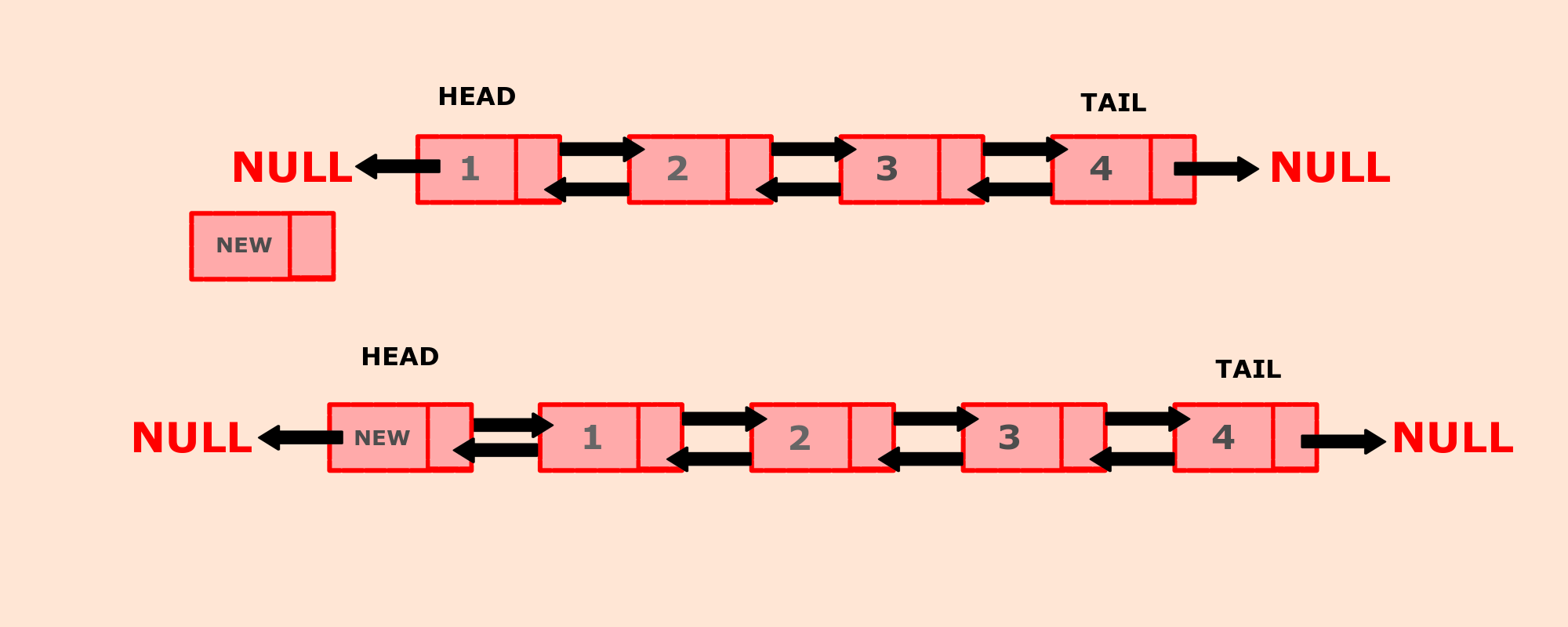
Credit: www.javatpoint.com
Implementation in Python
Let’s begin by defining a Node class that will represent each node in our doubly linked list:
class Node:
def __init__(self, data=None, prev=None, next=None):
self.data = data
self.prev = prev
self.next = next
Now, let’s create the DoublyLinkedList class, which will manage our doubly linked list:
class DoublyLinkedList:
def __init__(self):
self.head = None
The Append Method
One of the fundamental operations in a linked list is appending a new node to the end. Let’s define an append method in our DoublyLinkedList class:
def append(self, data):
new_node = Node(data)
if self.head is None:
self.head = new_node
else:
current_node = self.head
while current_node.next:
current_node = current_node.next
current_node.next = new_node
new_node.prev = current_node
The append method first checks if the head is empty. If it is, it assigns the new node as the head. Otherwise, it traverses the list until it reaches the last node and appends the new node after it. The new node’s previous reference is set to the current node.
Testing the Append Method
Let’s create an instance of the DoublyLinkedList class and test the append method:
# Creating a DoublyLinkedList instance
my_list = DoublyLinkedList()
# Appending new nodes
my_list.append(10)
my_list.append(20)
my_list.append(30)
my_list.append(40)
# Displaying the list
current_node = my_list.head
while current_node:
print(current_node.data)
current_node = current_node.next
The output should be:
10
20
30
40
As seen in the code snippet above, we create a DoublyLinkedList instance and append four new nodes to it. Finally, we traverse the list and display the data of each node.
Frequently Asked Questions For Building A Doublylinkedlist In Python – – Append Method
How Does The Append Method Work In Python’s Doubly Linked List?
The append method in Python’s Doubly Linked List allows you to add a new node at the end of the list. By setting the next pointer of the last node to the new node, the new node becomes the last node in the list.
What Is The Advantage Of Using The Append Method In A Doubly Linked List?
The append method enables efficient addition of new nodes to the end of the list. This advantage is particularly useful when the list is frequently updated, as it avoids the need to traverse the entire list to reach the end node.
Can The Append Method Be Used To Add A New Node Anywhere Within The Doubly Linked List?
No, the append method is specifically designed to add a new node at the end of the list. If you want to add a new node at a different position, you would need to use other methods such as insert or add, which are specifically implemented for that purpose.
How Do I Implement The Append Method In Python’s Doubly Linked List?
To implement the append method, you first need to check if the list is empty. If it is, you simply assign the new node as the head. Otherwise, you traverse the list to reach the last node and set its next pointer to the new node.
Conclusion
In this article, we learned about building a doubly linked list in Python and focusing on the append method. A doubly linked list is a flexible data structure that allows for efficient insertion and deletion operations at both ends of the list. The append method enables us to add new nodes to the end of the list in a straightforward manner.