What is the Meaning of Syntax Error in Python: A Quick Guide
A syntax error in Python occurs when the code violates the language’s grammatical rules. It prevents the program from executing.
Python is a popular programming language known for its simplicity and readability. Syntax errors can be frustrating for beginners and experienced developers alike. These errors arise from incorrect use of the language’s structure, such as missing colons, parentheses, or incorrect indentation.
Detecting and fixing syntax errors is crucial for the smooth execution of your code. Understanding common syntax mistakes can save time and effort. This guide will help you identify and resolve these errors, ensuring your Python code runs flawlessly. Dive in to learn more about avoiding syntax pitfalls in your Python projects.
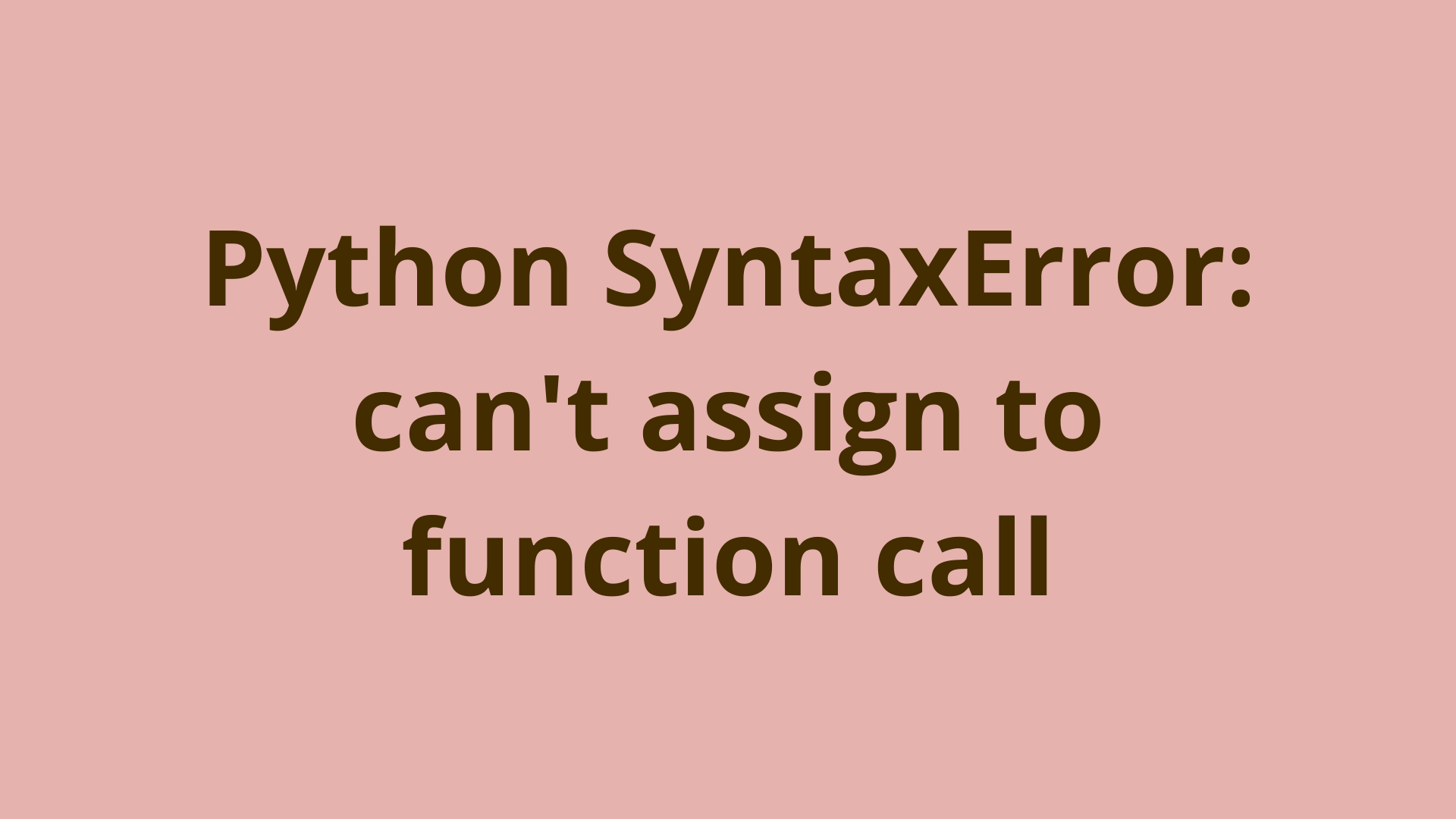
Defining Syntax Error
In Python programming, syntax errors occur when the code structure is incorrect. These errors prevent the code from executing. Understanding syntax errors is crucial for debugging code.
Basic Concept
A syntax error happens if the Python interpreter finds invalid code. The interpreter reads the code line by line and checks for correct syntax. If the syntax is wrong, it raises a SyntaxError.
For example, missing a colon in an if statement:
if x == 10
print("x is 10")
The correct syntax is:
if x == 10:
print("x is 10")
Common Causes
Several common mistakes lead to syntax errors in Python:
- Missing colons at the end of control structures (if, for, while).
- Unmatched parentheses or brackets.
- Incorrect indentation which is crucial in Python.
- Incorrect use of quotes in string literals.
- Misspelled keywords or function names.
Here is a table showing common mistakes and their corrections:
Common Mistake | Correction |
---|---|
if x == 10 | if x == 10: |
print("Hello World) | print("Hello World") |
def my_function() | def my_function(): |
[1, 2, 3 | [1, 2, 3] |
Understanding and fixing syntax errors will make your code run smoothly. These common causes can help you debug your Python programs effectively.
Examples Of Syntax Errors
Syntax errors are common in Python programming. They occur when the code structure is incorrect. These errors prevent the program from running. Understanding syntax errors can help you debug your code faster. Here are some examples of syntax errors you might encounter.
Missing Colons
One common syntax error is missing colons. In Python, colons are used in various statements.
For example, missing a colon in a function definition causes an error:
def greet(name)
print(f"Hello, {name}")
The correct syntax includes a colon after the function definition:
def greet(name):
print(f"Hello, {name}")
Another example is missing a colon in a for loop:
for i in range(5)
print(i)
The corrected version with a colon:
for i in range(5):
print(i)
Misplaced Parentheses
Another frequent syntax error involves misplaced parentheses. Parentheses must match in pairs.
For example, a misplaced parenthesis in a print statement causes an error:
print("Hello, world!"
The correct syntax includes closing the parenthesis:
print("Hello, world!")
Misplaced parentheses can also occur in function calls:
result = sum(1, 2, 3
The corrected version with matching parentheses:
result = sum(1, 2, 3)
Properly matching parentheses is crucial to avoid these errors.
Detecting Syntax Errors
Understanding how to detect syntax errors in Python is essential. Syntax errors occur when the Python interpreter finds incorrect code. This stops the code from running. Knowing how to identify these errors can save time and frustration.
Error Messages
Python provides clear error messages when syntax errors occur. These messages help identify the problem in the code. For example, if you forget a colon in a loop, Python will point it out.
for i in range(10)
print(i)
# Output: SyntaxError: invalid syntax
In this example, the error message indicates a missing colon. It suggests where the mistake is located.
Line Numbers
Each error message includes line numbers. This shows exactly where the error is. Let’s look at another example:
def greet()
print("Hello, World!")
# Output: SyntaxError: invalid syntax on line 1
The error message points to line 1. This helps you quickly find and fix the issue.
Using error messages and line numbers can greatly improve your coding efficiency. It makes debugging less stressful and more straightforward.
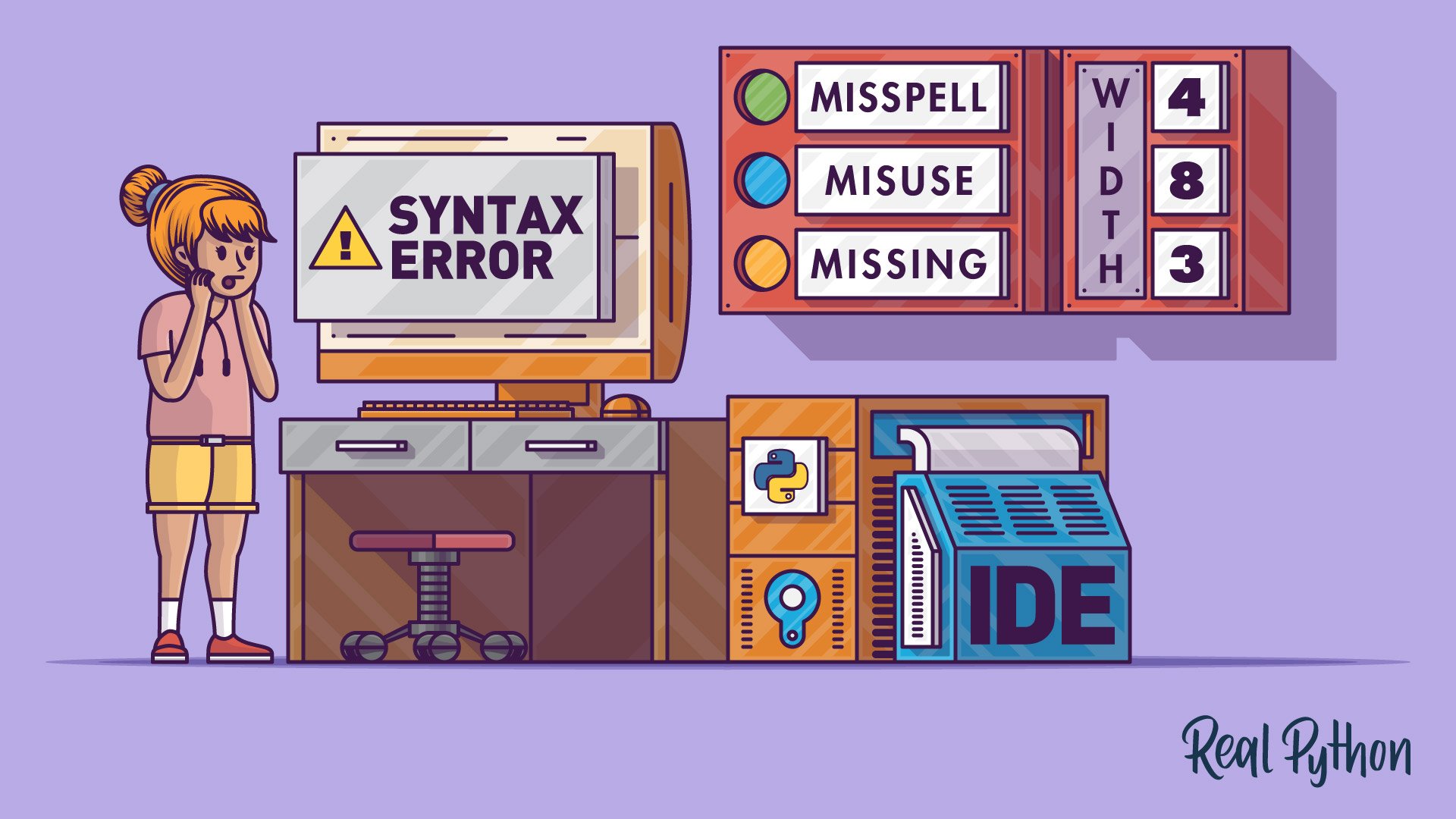
Credit: realpython.com
Common Syntax Mistakes
Syntax errors in Python can be frustrating. They happen when the code is not written correctly. Understanding common syntax mistakes helps in writing better code.
Indentation Issues
Python uses indentation to define code blocks. Incorrect indentation leads to syntax errors. Here are some common mistakes:
- Using tabs and spaces together.
- Incorrect indentation of loops and functions.
Example of incorrect indentation:
def my_function():
print("Hello World")
Correct indentation:
def my_function():
print("Hello World")
Incorrect Keywords
Using incorrect keywords also causes syntax errors. Here are some common mistakes:
- Misspelling keywords like def, if, and else.
- Using reserved words as variable names.
Example of incorrect keyword usage:
if x = 5:
print("x is 5")
Correct usage:
if x == 5:
print("x is 5")
Understanding these common mistakes can help avoid syntax errors. This makes coding in Python easier and more fun.
Tools For Syntax Checking
Understanding the meaning of syntax errors in Python is crucial. These errors occur when the code does not follow the correct format. To help catch these errors early, various tools can be used for syntax checking.
Ides And Editors
Many Integrated Development Environments (IDEs) and code editors have built-in syntax checkers. These tools highlight syntax errors as you type. This helps you fix issues quickly. Popular IDEs for Python include:
- PyCharm: Offers real-time error highlighting and suggestions.
- VS Code: Supports extensions that check Python syntax.
- Spyder: Comes with an integrated error checker.
Using these tools can save time and reduce frustration. They provide instant feedback, making coding easier and more fun.
Linting Tools
Linting tools are another way to check syntax. These tools not only find syntax errors but also enforce coding standards. They help maintain code quality and readability. Some popular linting tools for Python include:
- Pylint: Analyzes your code for errors and enforces a coding standard.
- Flake8: Combines several tools to check the style and quality of your code.
- Pyflakes: Focuses on finding syntax errors and unused imports.
Using linting tools helps keep your code clean and error-free. They integrate with most IDEs and editors, providing seamless syntax checking.
Fixing Syntax Errors
Syntax errors in Python can be frustrating, especially for beginners. They occur when the code doesn’t follow the rules of the Python language. These errors can prevent your code from running. Fixing them is crucial for your program to work correctly.
Step-by-step Debugging
Debugging is the process of finding and fixing errors in your code. Follow these steps to fix syntax errors:
- Read the error message: Python provides a message that helps you find the error.
- Check the line number: The message shows where the error is located.
- Review your code: Look at the code around the error message.
- Fix the error: Correct the mistake and save your changes.
- Run your code again: Check if the error is resolved.
Using Documentation
Python documentation is a valuable resource for fixing syntax errors. It provides information about the correct syntax and common mistakes. Here’s how to use it:
- Search for the error: Look up the specific error message in the documentation.
- Read examples: Look at code examples to understand the correct syntax.
- Follow guidelines: Follow the guidelines provided to fix the error.
Using documentation helps you learn and avoid future mistakes.
Here’s a simple example of a syntax error and how to fix it:
# This code has a syntax error
print("Hello World
The error message might say “EOL while scanning string literal”. It means the string is not closed properly. To fix it:
# Corrected code
print("Hello World")
This small change fixes the syntax error and your code will run.
Preventing Syntax Errors
Understanding the meaning of syntax errors in Python is crucial. These errors happen when the code breaks the rules of Python’s language. Preventing syntax errors can save you time and frustration.
Writing Clean Code
Writing clean code is a good way to avoid syntax errors. Clean code is easy to read and understand. Follow these tips to write clean code:
- Use proper indentation: Python relies on indentation to define blocks of code.
- Keep lines short: Long lines are hard to read and understand.
- Use meaningful names: Name your variables and functions clearly.
- Comment your code: Add comments to explain complex parts.
Regular Practice
Regular practice helps prevent syntax errors. The more you code, the better you get. Follow these steps to improve through practice:
- Code every day: Make coding a daily habit.
- Review your code: Check your code for errors regularly.
- Learn from mistakes: Understand and fix your errors.
- Join coding communities: Share and discuss code with others.
Tip | Explanation |
---|---|
Indentation | Use spaces or tabs consistently. |
Short Lines | Keep lines under 80 characters. |
Meaningful Names | Choose descriptive names for variables and functions. |
Comments | Add comments to explain tricky parts. |
By following these tips, you can write better code. This helps prevent syntax errors in Python.

Credit: stackoverflow.com
Frequently Asked Questions
What Is A Syntax Error In Python?
A syntax error in Python occurs when the code structure is incorrect. It means the code doesn’t follow the language’s rules.
How To Identify Syntax Errors In Python?
Python’s interpreter highlights syntax errors with specific messages. These messages often include the line number of the error.
What Causes Syntax Errors In Python?
Syntax errors in Python are caused by incorrect code formatting. Common issues include missing colons, parentheses, or incorrect indentation.
Can Syntax Errors Be Avoided?
Yes, syntax errors can be avoided by carefully writing and reviewing your code. Using a code editor with syntax highlighting also helps.
Conclusion
Understanding syntax errors in Python is crucial for effective coding. These errors disrupt program execution. Identifying and fixing them ensures smooth performance. Pay attention to details and follow Python’s syntax rules. Proper practice and patience will help you master this aspect.
Happy coding!