How Much Time to Learn Python to Get a Job: Quick Guide
You can learn Python and get a job in 3 to 6 months with consistent effort. This timeframe varies depending on prior experience and dedication.
Python is a versatile, beginner-friendly programming language widely used in various industries. With its easy-to-read syntax and robust libraries, Python is ideal for both beginners and experienced programmers. Companies value Python skills for web development, data analysis, artificial intelligence, and automation.
Dedicating a few hours each day to learning Python can significantly boost your job prospects. Online courses, coding bootcamps, and practice projects can accelerate your learning process. Mastering Python basics and building a portfolio of projects will enhance your employability. The key is consistent practice and applying your knowledge to real-world scenarios.

Credit: www.interviewkickstart.com
Introduction To Python
Python is a popular programming language. It is known for its simplicity. Python is used in many fields like web development, data science, and artificial intelligence. It is a great language for beginners. Python’s syntax is easy to understand and write.
Why Choose Python?
Python is versatile. You can use it for web development, data analysis, and even artificial intelligence. It has a large community. This means you can find help easily. There are many libraries and frameworks. They make coding faster and easier.
- Web Development: Flask, Django
- Data Science: Pandas, NumPy
- Machine Learning: TensorFlow, Keras
Python In The Job Market
Python is in high demand in the job market. Many companies look for Python developers. Python skills can lead to various roles. Some of them include:
- Web Developer
- Data Scientist
- Machine Learning Engineer
Python’s popularity is growing. This means more job opportunities. Learning Python can help you get a good job. It is a valuable skill to have.
Role | Average Salary |
---|---|
Web Developer | $70,000 per year |
Data Scientist | $90,000 per year |
Machine Learning Engineer | $110,000 per year |
Python is a great choice for a career in tech. Start learning Python today!
Learning Basics
Starting with Python can be exciting. The basics are easy to learn. You will need to understand a few key concepts. These include syntax, variables, and control structures.
Syntax And Variables
Python syntax is simple and clear. This makes it beginner-friendly. Here is an example:
print("Hello, World!")
The print
function displays text. In Python, variables do not need a declaration. You can create a variable by assigning a value:
x = 5
y = "Hello, World!"
Variables can store different types of data. These include numbers and strings. Python uses dynamic typing. This means the type of a variable is determined at runtime.
Control Structures
Control structures guide the flow of your program. Python has several types:
- If statements: Used for decision-making.
- Loops: Used for repeating actions.
Here is an example of an if statement:
x = 10
if x > 5:
print("x is greater than 5")
Python supports two main types of loops: for loops and while loops.
Example of a for loop:
for i in range(5):
print(i)
This loop prints numbers from 0 to 4. Next, an example of a while loop:
i = 0
while i < 5:
print(i)
i += 1
This loop also prints numbers from 0 to 4.
Intermediate Concepts
Understanding intermediate concepts in Python can accelerate your path to a job. This section covers Functions and Modules and Data Structures. These are key for tackling real-world programming tasks.
Functions And Modules
Functions are reusable pieces of code. They make your program organized. Here is a simple function:
def greet(name):
return f"Hello, {name}"
Modules are files with Python code. They help divide your code into manageable parts. To use a module, employ the import
statement:
import math
print(math.sqrt(9))
Learning to use functions and modules improves code readability. It also makes debugging easier. Mastering these can set you apart in job interviews.
Data Structures
Data structures are ways to store and organize data. Python offers several built-in data structures:
- Lists: Ordered collections, mutable.
- Tuples: Ordered collections, immutable.
- Dictionaries: Key-value pairs, mutable.
- Sets: Unordered collections, unique elements.
Here is a table to compare these data structures:
Data Structure | Ordered | Mutable | Unique Elements |
---|---|---|---|
List | Yes | Yes | No |
Tuple | Yes | No | No |
Dictionary | No | Yes | No |
Set | No | Yes | Yes |
Knowing these data structures helps in optimizing your code. It also makes data manipulation easier. Employers value candidates with strong knowledge of data structures.
Advanced Topics
Mastering Python’s advanced topics is crucial for landing a top-tier job. These subjects can set you apart from other candidates. Let’s delve into two essential advanced topics: Object-Oriented Programming and Libraries and Frameworks.
Object-oriented Programming
Object-Oriented Programming (OOP) is a fundamental concept in Python. It allows you to create classes and objects. Understanding OOP helps in writing efficient and reusable code. Key concepts include:
- Classes and Objects: Create templates (classes) and instances (objects).
- Inheritance: Derive new classes from existing ones.
- Polymorphism: Use methods in different ways.
- Encapsulation: Hide internal states and functionalities.
Here is a basic example of OOP in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def speak(self):
return "Woof!"
dog = Dog("Buddy")
print(dog.speak()) # Output: Woof!
Libraries And Frameworks
Python has a vast range of libraries and frameworks. Mastering these can significantly enhance your skill set. Some popular ones include:
Category | Library/Framework | Description |
---|---|---|
Web Development | Django | A high-level web framework for rapid development. |
Data Science | Pandas | Data manipulation and analysis. |
Machine Learning | TensorFlow | Comprehensive ML library for building models. |
Automation | Selenium | Web browser automation tool. |
Learning these tools can make you more employable. They allow you to tackle complex problems with ease.
Practical Applications
Learning Python opens doors to many practical applications. You can build projects, contribute to open source, and gain real-world experience. These activities make you job-ready faster.
Building Projects
Building projects is key to mastering Python. Start with small projects to understand the basics. Gradually, tackle more complex tasks. Here are some ideas:
- Create a to-do list app
- Build a web scraper
- Develop a simple game
Each project improves your skills and showcases your abilities. Potential employers love seeing real-world applications.
Contributing To Open Source
Contributing to open source projects boosts your learning. It also helps you connect with other developers. GitHub is a great place to start. You can:
- Find projects that interest you
- Read the documentation and issues
- Submit pull requests with your contributions
Open source contributions enhance your resume. They show your commitment to the programming community. Employers value these contributions highly.
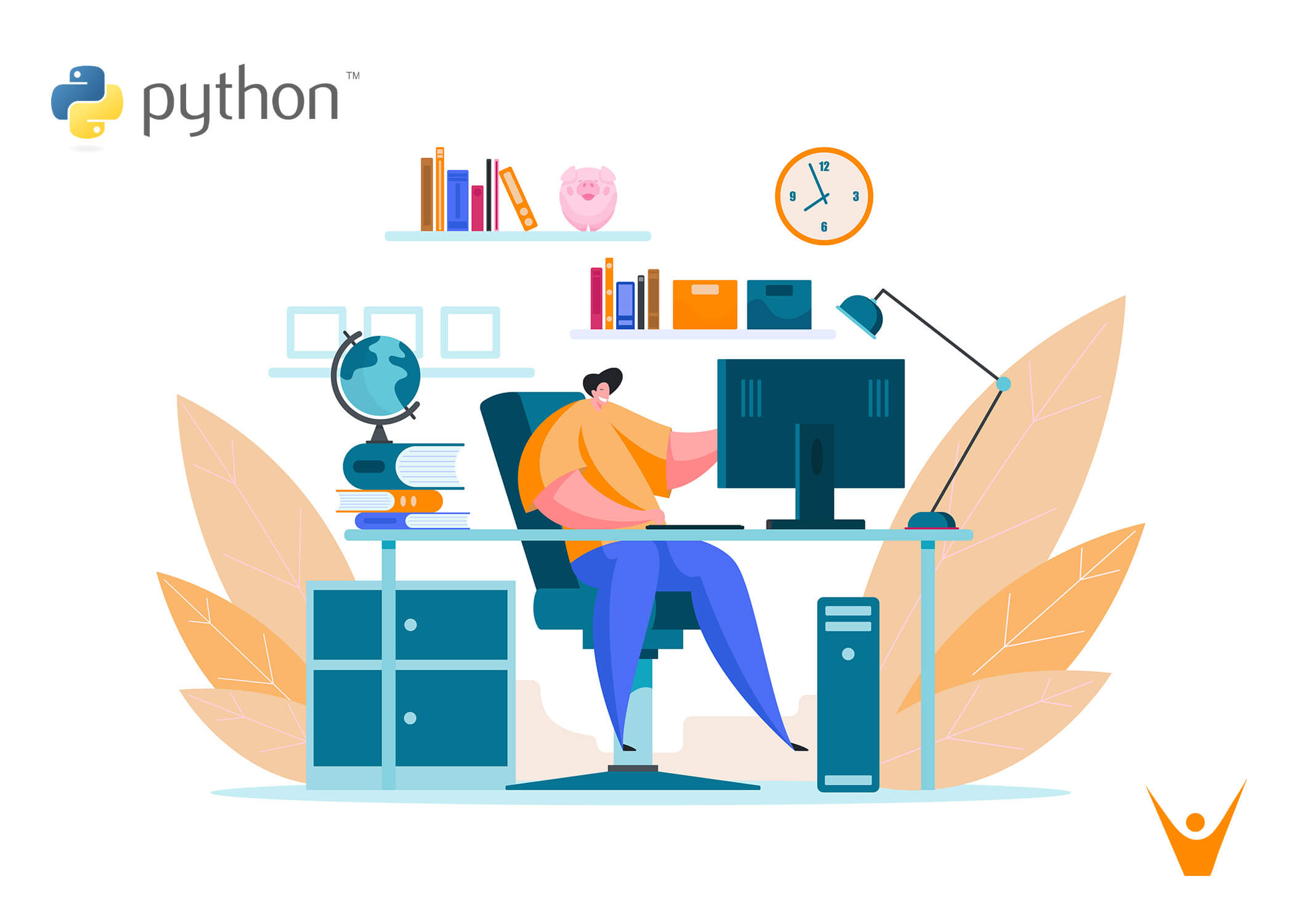
Credit: favtutor.com
Job Readiness
Learning Python can be quick, but job readiness takes more effort. It involves building a portfolio, preparing for interviews, and mastering the language.
Crafting A Portfolio
To get a job, you need a strong portfolio. Showcase your projects and skills clearly.
- Include various types of projects.
- Use GitHub to display your code.
- Write clear documentation for each project.
Focus on quality over quantity. Ensure your code is clean and well-organized.
Here are some project ideas:
- Web applications using Flask or Django.
- Data analysis projects with Pandas and NumPy.
- Automation scripts for daily tasks.
Preparing For Interviews
Interviews test your skills and knowledge. Practice common Python questions and algorithms.
Topic | Example Question |
---|---|
Data Structures | Explain the difference between a list and a tuple. |
Algorithms | How do you reverse a string in Python? |
Libraries | What is Pandas used for? |
Don’t forget soft skills. Practice explaining your code and thought process.
Mock interviews can help a lot. Get feedback and improve your weak areas.
Time Estimates
Understanding how much time it takes to learn Python for a job is crucial. This section will break down time estimates based on different learning paths and study modes.
Learning Paths
There are various learning paths to master Python. Each path has different time requirements. Here’s a breakdown:
Learning Path | Estimated Time |
---|---|
Self-Paced Online Courses | 6-12 months |
Bootcamps | 3-6 months |
University Degree | 3-4 years |
Self-Paced Online Courses: These allow flexibility. Learners can study at their own pace. Most people take about six months to a year.
Bootcamps: Intense and focused. Bootcamps usually last between three to six months. They provide a fast track to learning Python.
University Degree: A traditional path. A degree usually takes three to four years. It offers a comprehensive education in computer science.
Full-time Vs Part-time Study
Time to learn Python also depends on study commitment. Here’s a comparison:
- Full-Time Study: 4-8 hours per day, 5 days a week. This is the quickest way. It can take 3-6 months to get job-ready.
- Part-Time Study: 1-2 hours per day, 5 days a week. This is more flexible. It usually takes 6-12 months to become proficient.
Full-Time Study: Faster results. Dedicate 4-8 hours daily. You can be job-ready in 3-6 months.
Part-Time Study: More flexible. Study 1-2 hours daily. It takes 6-12 months to learn Python well.
Keep your goals and schedule in mind. Choose a path that fits your needs. Consistency is key to mastering Python.
Additional Resources
Learning Python to get a job can be a rewarding journey. To make this journey easier, you can use many additional resources. These resources will help you learn faster and better.
Online Courses
Online courses offer structured learning paths. They provide you with hands-on experience. Here are some popular platforms:
- Coursera: Offers courses from top universities.
- Udemy: Features affordable courses with lifetime access.
- edX: Provides free courses with the option to pay for certificates.
These platforms often include video tutorials, quizzes, and assignments. This helps you practice and test your knowledge.
Books And Tutorials
Books and tutorials are great for in-depth learning. They allow you to go at your own pace. Here are some recommended books:
- “Automate the Boring Stuff with Python” by Al Sweigart: Good for beginners.
- “Python Crash Course” by Eric Matthes: A hands-on, project-based book.
- “Learning Python” by Mark Lutz: Comprehensive and detailed.
Online tutorials are also very helpful. Websites like Real Python and W3Schools offer many tutorials. These tutorials are free and easy to follow.
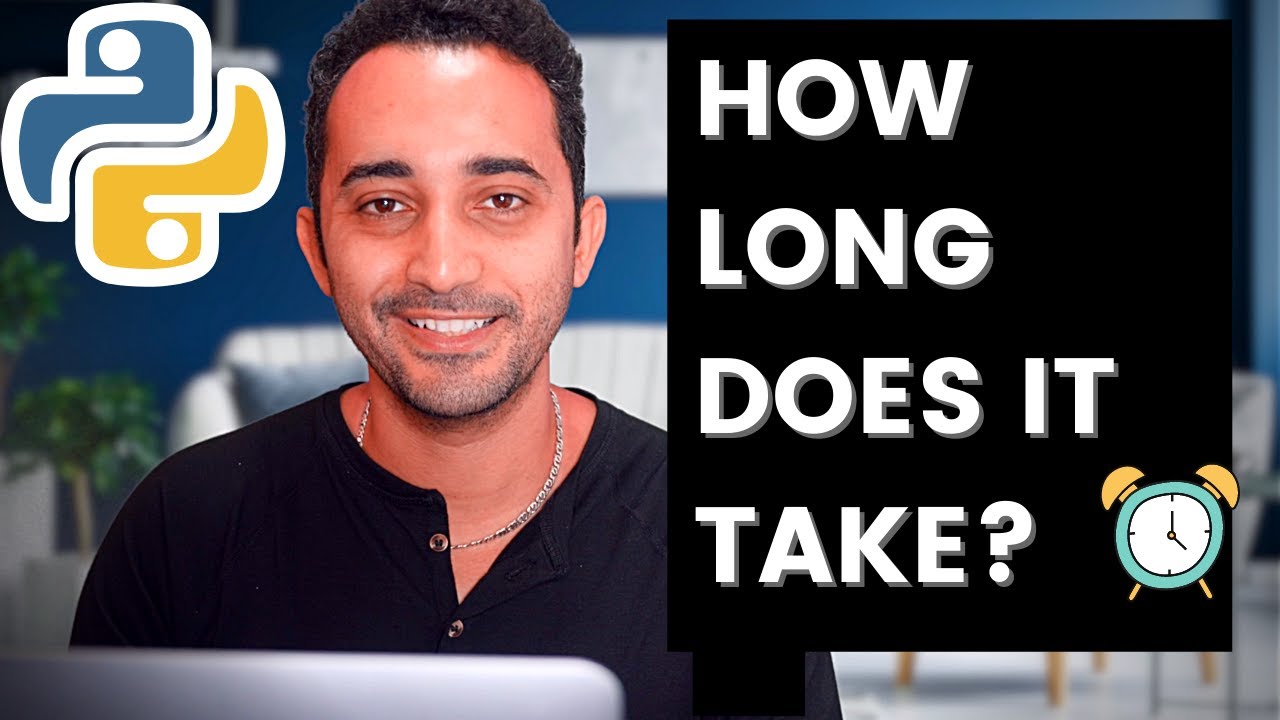
Credit: www.youtube.com
Frequently Asked Questions
How Long Does It Take To Learn Python For A Job?
Learning Python for a job can take 3-6 months. This depends on your dedication and prior programming experience. Practice and real-world projects are crucial.
Can I Get A Job With Basic Python Skills?
Yes, you can get entry-level jobs with basic Python skills. Focus on learning fundamentals and building small projects. Continuous learning is important.
What Python Skills Are Needed For A Job?
For a job, you need to understand Python basics, data structures, and libraries. Additionally, knowledge of frameworks like Django or Flask is beneficial.
Is Python Enough To Get A Job?
Python alone can be enough to get a job. However, knowing additional skills like SQL, and version control can improve your chances.
Conclusion
Mastering Python for a job varies by individual dedication. Typically, it takes a few months of focused learning. Regular practice and real-world projects boost your skills. Online courses and coding bootcamps can expedite the process. With persistence, you’ll be ready to land a Python job soon.