Run Part of a Script With a Different Version of Python
When it comes to running Python scripts, having the right version of Python installed is crucial. However, sometimes you may encounter a situation where you need to run only a specific part of a script with a different version of Python. In this article, we will explore different methods to achieve this.
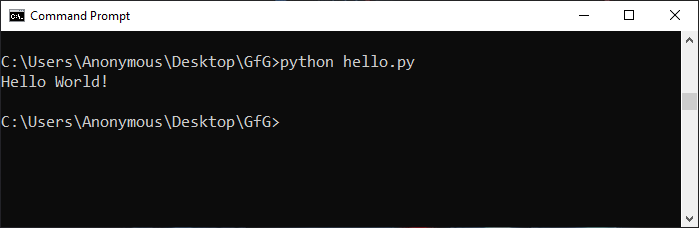
Credit: www.geeksforgeeks.org

Credit: generalassemb.ly
Method 1: Using a Python Version Manager
One of the easiest ways to run part of a script with a different Python version is by utilizing a version manager such as conda or pyenv.
If you have conda installed, you can create a separate environment using the desired Python version with the following command:
conda create -n myenv python=3.7
This command will create a new environment named “myenv” with Python version 3.7. You can activate this environment by running:
conda activate myenv
Once activated, you can run your script in this specific environment, and it will use the Python version specified.
If you prefer to use pyenv, you can install the desired Python version using the following command:
pyenv install 3.7.0
This will install Python version 3.7.0. Then you can create a virtual environment with the specified Python version using:
pyenv virtualenv 3.7.0 myenv
Activate the virtual environment:
pyenv activate myenv
Now you can run your script within the specified virtual environment, allowing you to utilize the desired Python version.
Method 2: Using Shebang Line
The Shebang line is a special comment at the beginning of a script that specifies an interpreter. You can use this line to specify the desired Python version for a specific part of your script.
For example, if you have Python 3.6 installed and want to run a specific function using Python 3.7, you can add the following Shebang line before the function:
#!/usr/bin/env python3.7
By adding this Shebang line, the script will be executed using the specified Python version, even if your default version is different.
Method 3: Running Part of the Script As a Subprocess
If you have a more complex scenario where you need to run a specific part of a script with a different Python version and capture or use the output in your main script, you can use the subprocess module.
Here is an example of how you can achieve this:
import subprocess
# Run Python script with a different version
result = subprocess.check_output(['python3.7', 'your_script.py'], universal_newlines=True)
# Use the result in your main script
print(result)
By utilizing the subprocess module, you can execute a separate Python script with a specific version while still capturing and utilizing the output within your main script.
These methods provide you with flexibility when it comes to running part of a script with a different version of Python. Choose the method that best suits your needs and ensures your script runs smoothly with the desired Python version.
Frequently Asked Questions Of Run Part Of A Script With A Different Version Of Python
How Do I Run A Script With A Different Version Of Python?
To run a script with a different version of Python, you can specify the specific version by using the command line interface or virtual environments.
What Are The Advantages Of Running A Script With A Different Version Of Python?
Running a script with a different version of Python allows you to leverage new features and improvements, ensure compatibility with specific libraries, and test the script’s performance with different versions.
Can I Install Multiple Versions Of Python On My System?
Yes, you can install multiple versions of Python on your system. Using tools like pyenv or virtual environments can help manage different Python versions efficiently.
How Can I Check The Installed Python Versions On My System?
To check the installed Python versions, open the terminal or command prompt and type “python –version” or “python3 –version” to display the installed Python version.