What is the Difference Between a Syntax, Logic, and Runtime Error in Python?
A syntax error occurs when Python cannot understand the code due to incorrect structure. A logic error produces incorrect results, while a runtime error crashes the program during execution.
Understanding these three types of errors is crucial for effective debugging. Syntax errors are often the easiest to spot. They arise from typos or incorrect use of Python’s syntax. Logic errors, on the other hand, are more insidious. They occur when the code runs without crashing but produces incorrect results.
Runtime errors happen when the program encounters an issue that prevents it from continuing, like dividing by zero or accessing a nonexistent file. Knowing how to identify and fix these errors can save time and enhance coding efficiency.
Introduction To Python Errors
Programming in Python is fun and powerful. Yet, errors can occur. Understanding different types of errors is crucial. This knowledge helps in troubleshooting. Let’s explore the main types of errors in Python.
Common Error Types
Errors in Python generally fall into three categories. These are syntax errors, logic errors, and runtime errors.
Error Type | Description | Example |
---|---|---|
Syntax Error | Occurs when code breaks Python’s syntax rules. | print("Hello World missing closing parenthesis |
Logic Error | Code runs but produces incorrect results. | if x = 10: instead of if x == 10: |
Runtime Error | Occurs while the program is running. | print(10/0) division by zero |
Importance Of Error Handling
Handling errors is essential. It makes programs robust and user-friendly. Proper error handling ensures programs do not crash unexpectedly. It also helps in debugging and maintaining the code.
- Prevents unexpected crashes
- Helps identify and fix bugs
- Improves user experience
Here is a sample code demonstrating error handling:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
In this code, the try
block attempts division. The except
block catches the ZeroDivisionError. This prevents the program from crashing.
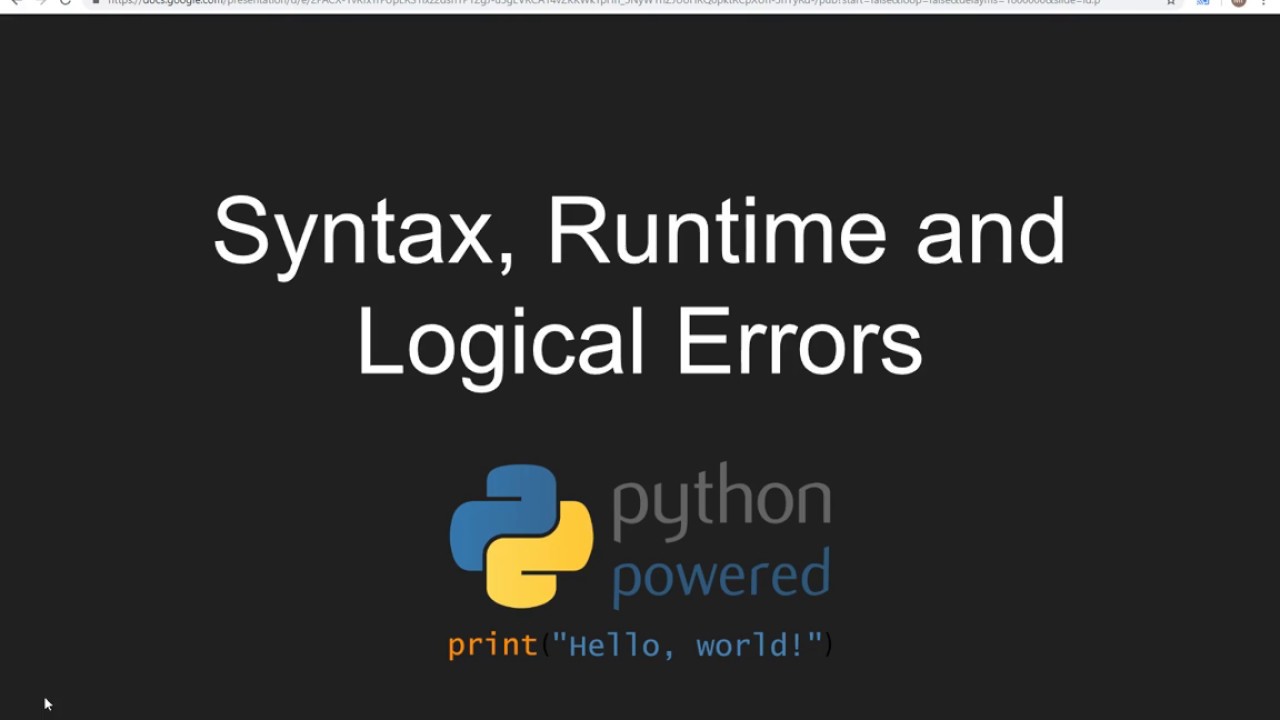
Credit: m.youtube.com
Syntax Errors
Syntax errors in Python occur when the code violates the language’s grammatical rules, preventing execution. They differ from logic and runtime errors, which involve incorrect algorithms and issues during program execution, respectively.
Syntax errors are the most common type of error in Python. They occur when the code does not follow the correct structure or rules of the language. This type of error is detected by the Python interpreter during the parsing stage, and it prevents the code from running.
Definition
A syntax error happens when the code has incorrect syntax. This means that the code does not conform to the rules of Python language. The interpreter catches these errors before the program runs.
Common Causes
- Missing colons (:) at the end of control structures
- Incorrect indentation
- Unmatched parentheses, brackets, or braces
- Incorrect or missing quotation marks
Examples And Fixes
Example | Cause | Fix |
---|---|---|
if x == 10 print("x is 10") |
Missing colon (:) after if statement | if x == 10: |
for i in range(10) |
Missing colon (:) after for statement | for i in range(10): |
print("Hello World) |
Unmatched quotation mark | print("Hello World") |
Understanding syntax errors is crucial. They are the first type of error you’ll likely encounter. Fixing them is often straightforward, but spotting them quickly can save much time.
Logic Errors
Logic errors in Python occur when the code runs without crashing but produces incorrect results. These errors are different from syntax and runtime errors because they don’t stop the program from running. Instead, they cause the program to behave unexpectedly.
Definition
A logic error happens when the code you write doesn’t do what you intended. The program runs, but the output is incorrect. These errors are difficult to find because the code looks correct and runs without crashing.
Common Causes
Logic errors often arise from:
- Incorrect use of operators
- Wrong variable assignments
- Faulty conditionals in if-else statements
- Mistakes in loops
Examples And Fixes
Here are some typical logic errors and how to fix them:
Error Type | Example | Fix |
---|---|---|
Incorrect use of operators | result = 5 + 3 2 |
Use parentheses to clarify order: result = (5 + 3) 2 |
Wrong variable assignments | x = y + 1 instead of y = x + 1 |
Check the variable names and logic. |
Faulty conditionals | if (age > 18): eligible = True |
Ensure all conditions are correct. |
Mistakes in loops | for i in range(1, 10): print(i) |
Check loop bounds: for i in range(1, 11): print(i) |
By paying attention to these common causes and fixes, you can avoid logic errors. Always test your code thoroughly to ensure it behaves as expected.

Credit: pediaa.com
Runtime Errors
Understanding runtime errors in Python is crucial for smooth programming. These errors occur while your program is running. They can cause your program to crash or behave unexpectedly.
Definition
Runtime errors happen during the execution of a program. They are not caught by the compiler. They often cause the program to stop abruptly. These errors are also called exceptions.
Common Causes
- Division by zero
- File not found
- Out of memory
- Invalid type operations
Examples And Fixes
# This will cause a runtime error
number = 10 / 0
Fix: Use a try-except block to handle the exception.
try:
number = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
# This will cause a runtime error
file = open("non_existent_file.txt")
Fix: Check if the file exists before opening.
import os
if os.path.exists("non_existent_file.txt"):
file = open("non_existent_file.txt")
else:
print("File not found!")
# This will cause a runtime error
number = "123" + 456
Fix: Ensure that types are compatible.
number = "123" + str(456)
Comparing Error Types
In Python, errors can be categorized into three main types: syntax errors, logic errors, and runtime errors. Each type of error affects code execution differently and requires unique strategies for prevention and detection. Understanding these differences is crucial for efficient programming and debugging.
Impact On Code Execution
Syntax errors occur when the code does not follow Python’s grammar rules. The program will not run at all. These errors are detected by the Python interpreter during the parsing stage.
Logic errors happen when the code runs but produces incorrect results. The program executes without crashing, making these errors harder to identify.
Runtime errors occur while the program is running. They cause the program to crash, and are detected during the execution phase.
Error Type | Impact on Execution |
---|---|
Syntax Error | Code will not run |
Logic Error | Code runs but gives incorrect results |
Runtime Error | Code runs but crashes |
Ease Of Detection
Syntax errors are the easiest to detect. The Python interpreter highlights them directly, providing error messages that indicate the line and nature of the error.
Logic errors are more challenging to find. They do not produce error messages. Instead, they require careful code review and testing to identify incorrect outputs.
Runtime errors are easier to detect than logic errors but harder than syntax errors. These errors produce error messages during execution, indicating where the crash occurred.
- Syntax Error: Easy to detect
- Logic Error: Hard to detect
- Runtime Error: Moderate to detect
Strategies For Prevention
Preventing syntax errors involves writing clean, well-structured code. Using an Integrated Development Environment (IDE) with syntax highlighting and linting tools can help.
To avoid logic errors, implement thorough testing, including unit tests and integration tests. Code reviews and pair programming can also help identify logical flaws.
Preventing runtime errors requires handling exceptions properly. Use try-except blocks to catch and manage potential runtime issues gracefully.
- Syntax Errors: Use an IDE with syntax highlighting
- Logic Errors: Implement thorough testing
- Runtime Errors: Use try-except blocks for exception handling

Credit: slideplayer.com
Real-world Scenarios
Understanding the differences between syntax, logic, and runtime errors in Python is essential. Real-world scenarios help illustrate how these errors impact programs.
Case Studies
Let’s explore some real-world scenarios to understand these errors better.
Case Study 1: Syntax Error
A syntax error occurs due to incorrect code structure. For instance:
print "Hello, World!"
This code will raise a syntax error because the print statement lacks parentheses.
- Issue: Missing parentheses in the print function.
- Solution: Use
print("Hello, World!")
instead.
Case Study 2: Logic Error
A logic error produces incorrect results without crashing the program. For example:
def add_numbers(a, b):
return a - b
This function is intended to add two numbers but subtracts them instead.
- Issue: Incorrect operator used.
- Solution: Replace
a - b
witha + b
.
Case Study 3: Runtime Error
A runtime error occurs while the program is running. For instance:
numbers = [1, 2, 3]
print(numbers[5])
This code will raise an IndexError because index 5 does not exist.
- Issue: Accessing an out-of-range index.
- Solution: Ensure the index is within the list’s range.
Lessons Learned
Understanding these errors helps improve coding skills.
- Syntax Errors: Always check the code structure.
- Logic Errors: Validate the code’s logic flow.
- Runtime Errors: Handle exceptions gracefully.
Learning from these mistakes makes you a better programmer.
Tools And Techniques
Understanding the differences between syntax, logic, and runtime errors in Python is crucial. Knowing the right tools and techniques can save time and effort. This section will cover essential debugging tools and best practices to identify and resolve these errors efficiently.
Debugging Tools
Various tools can help identify syntax, logic, and runtime errors in Python. Below are some of the most effective ones:
- PyCharm: A popular IDE that provides robust debugging features.
- Visual Studio Code: Lightweight and highly customizable, ideal for debugging.
- PDB (Python Debugger): A built-in debugger for stepping through code.
- Linting Tools: Tools like Flake8 and Pylint help catch syntax errors early.
Best Practices
Adhering to best practices can minimize the occurrence of syntax, logic, and runtime errors. Here are some tips:
- Write clean and readable code. Use meaningful variable names.
- Break down your code into smaller functions. This makes debugging easier.
- Always include comments and docstrings to explain your code.
- Use version control like Git to keep track of changes.
- Regularly run unit tests to catch errors early.
Using these tools and best practices can significantly reduce errors in your Python code. They make debugging more manageable and efficient.
Frequently Asked Questions
What Is A Syntax Error In Python?
A syntax error occurs when Python code breaks language rules. This usually happens due to incorrect use of keywords, punctuation, or indentation.
How Does A Logic Error Differ From A Syntax Error?
A logic error occurs when the code runs but produces incorrect results. Unlike syntax errors, logic errors don’t prevent code from executing.
What Is A Runtime Error In Python?
A runtime error happens while the program is running. It causes the program to crash, often due to invalid operations or resource issues.
Can You Give Examples Of Syntax Errors?
Common syntax errors include missing colons, unmatched parentheses, and incorrect indentation. These errors prevent the code from running.
Conclusion
Understanding the differences between syntax, logic, and runtime errors in Python is crucial. It helps in efficient debugging and coding. Syntax errors are the easiest to spot. Logic errors require careful thought. Runtime errors often need thorough testing. Mastering these will enhance your programming skills and efficiency.