How Do You Count the Number of Rows in a Csv File in Python?
If you work with data in Python, you may often come across the need to count the number of rows in a CSV (Comma Separated Values) file. Whether you are analyzing a large dataset or preparing data for further processing, knowing how to count rows in a CSV file is an essential skill.
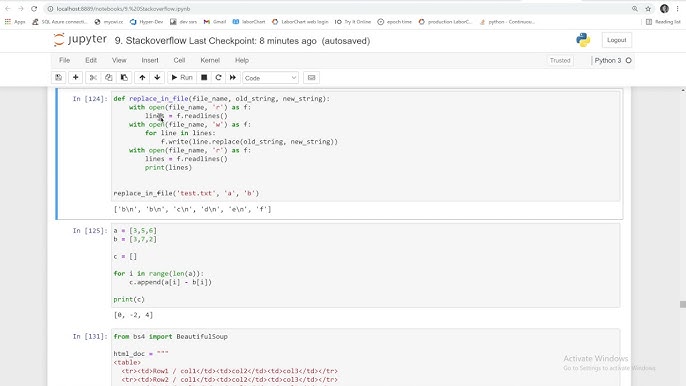
Credit: m.youtube.com
Using the CSV Module
Python provides a built-in module called csv that makes it easy to work with CSV files. To count the number of rows in a CSV file using this module, you can follow these steps:
Step 1: Import The Csv Module
First, you need to import the csv module in your Python script:
import csv
Step 2: Read The Csv File
Next, you need to open the CSV file using the open() function and create a csv.reader object:
with open('file.csv', 'r') as file: reader = csv.reader(file)
Replace file.csv with the path to your CSV file.
Step 3: Count The Rows
Now that you have the csv.reader object, you can simply iterate over it and count the rows:
row_count = 0 for row in reader: row_count += 1
The variable row_count will store the total number of rows in the CSV file.
Step 4: Print The Result
Finally, you can print the result:
print(f"The CSV file contains {row_count} rows.")
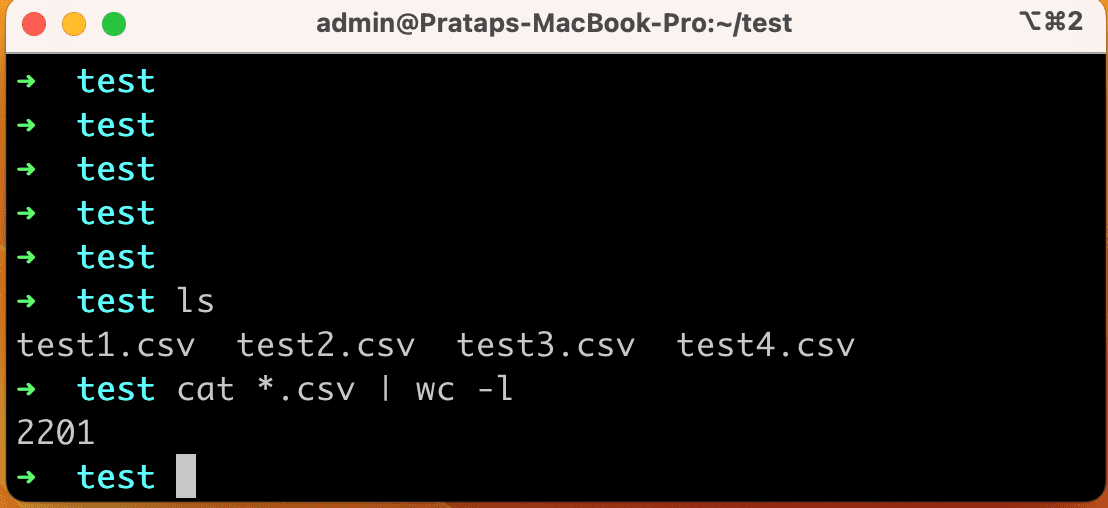
Credit: pratapsharma.com.np
Complete Example
Here’s the complete example for counting the number of rows in a CSV file:
import csv def count_rows(csv_file): with open(csv_file, 'r') as file: reader = csv.reader(file) row_count = 0 for row in reader: row_count += 1 return row_count filename = 'file.csv' rows = count_rows(filename) print(f"The CSV file '{filename}' contains {rows} rows.")
Make sure to replace file.csv with the path to your own CSV file.
Frequently Asked Questions Of How Do You Count The Number Of Rows In A Csv File In Python?
How Can I Count The Number Of Rows In A Csv File Using Python?
To count the number of rows in a CSV file using Python, you can utilize the `csv` module. First, you need to open the CSV file using the `open()` function and then create a CSV reader object using the `reader()` method from the `csv` module.
Finally, you can use the `len()` function to calculate the number of rows in the CSV file by passing the reader object as a parameter.
What Is The Python Code To Count The Rows In A Csv File?
To count the rows in a CSV file using Python, you can use the following code:
“`
import csv
with open(‘file.csv’, ‘r’) as csvfile:
reader = csv.reader(csvfile)
row_count = len(list(reader))
print(“The number of rows in the CSV file is:”, row_count)
“`
How Do I Count The Number Of Records In A Csv File Using Python?
To count the number of records in a CSV file using Python, you can employ the `csv` module. Begin by opening the CSV file with the `open()` function and then create a `csv. reader` object using the `reader()` method from the `csv` module.
You can iterate through the rows in the reader object and increment a counter variable for each record.
Is There A Built-in Function In Python To Count The Rows In A Csv File?
No, Python does not have a built-in function specifically dedicated to counting rows in a CSV file. However, you can achieve this by utilizing the `csv` module along with other functions and methods available in Python.
Conclusion
Counting the number of rows in a CSV file is a common task when working with data in Python. By using the csv module, you can easily read the CSV file and iterate over its rows to count them. Remember to import the module, read the file, count the rows, and print the result.
Now that you know how to count the rows in a CSV file in Python, you can efficiently handle large datasets or perform data preprocessing tasks with confidence.